Implement a feature flag in your application
Learn the basics of how to implement feature flags in your code.
When you add a feature flag to a story in Atono, you'll also need to include it in your codebase. Below, we provide sample code that demonstrates how to reference the feature flag and the environment it applies to.
Our feature flags are based on OpenFeature, an emerging standard for feature flagging that supports multiple platforms. For additional information, you can explore the OpenFeature SDK documentation.
1. Install the SDK
Run the following command in your project directory to install the Atono SDK for the platform you are using:
Client-side applications
npm install --save @atono-io/web-sdk
React applications
npm install --save @atono-io/react-sdk
Verify the installation
Run the following command to check that the installation was successful:
npm list @atono-io/web-sdk
or
npm list @atono-io/react-sdk
2. Add the flag to your code
Once you add a feature flag to a story, Atono will generate the following sample code that demonstrates how to implement the flag in your application.
Client-side example
// add the following to your app initialization (run once at startup)
const { Atono } = require('@atono-io/web-sdk');
const atono = Atono.fromEnvironmentKey('$ENV_KEY');
const featureFlags = await atono.getFeatureFlags();
// reference feature flags in your application logic as follows
if (featureFlags.getBooleanValue('flag_name', false)) {
// turn on the feature associated with your feature flag
}
You can copy the code from the Implement feature flag dialog. Paste the sample code in your application and use it to wrap the functionality you want to control with the feature flag.
React example
With the React SDK, wrap your application in the <AtonoProvider>
component and use the useFeatureFlag
hook to query flags in child components.
Root component:
import { AtonoProvider } from '@atono-io/react-sdk';
function RootComponent() {
return (
<AtonoProvider environmentKey="<your-environment-key>">
<ChildComponent />
</AtonoProvider>
);
}
Child component:
import { useFeatureFlag } from '@atono-io/react-sdk';
function ChildComponent() {
const isAmazingFeatureEnabled = useFeatureFlag()
.getBooleanValue('flag_name', false);
return isAmazingFeatureEnabled
? <div>Amazing feature is enabled π</div>
: <div>Amazing feature is not available π
</div>;
}
The React SDK automatically provides access to feature flags to any component wrapped in <AtonoProvider>
.
3. Reference your environment key
Next, you'll need to replace the $ENV_KEY
or <your-environment-key>
placeholder in the sample code with a reference to the environment variable that stores the environment key from Atono.
You can access your workspace's environment keys by clicking Environment keys in the Implement feature flag dialog or on the flag configuration screen:
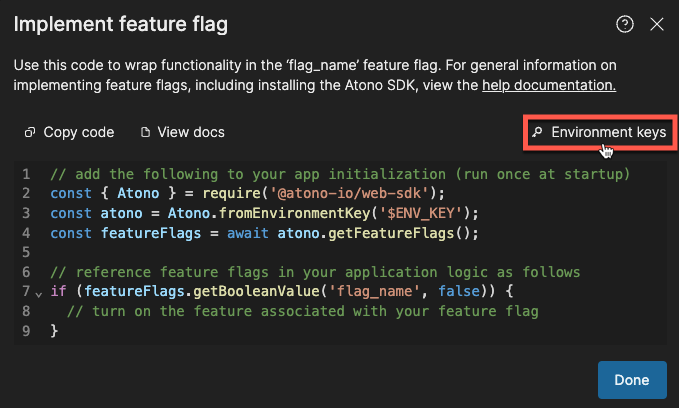
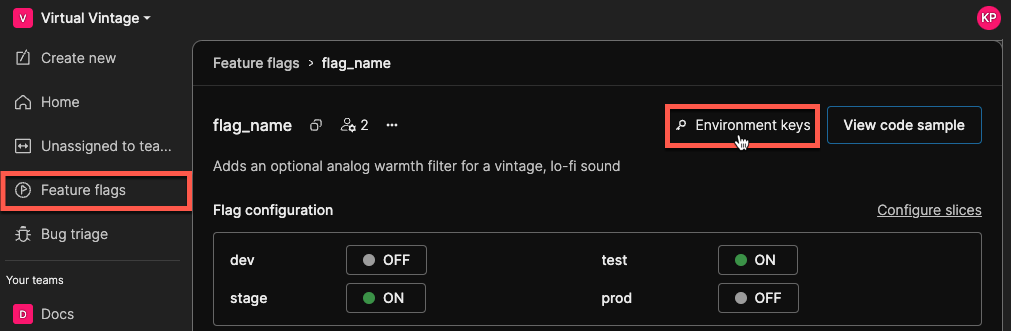
This will take you to the Environments page, where you can click the copy icon next to an environment to copy its key to your clipboard.
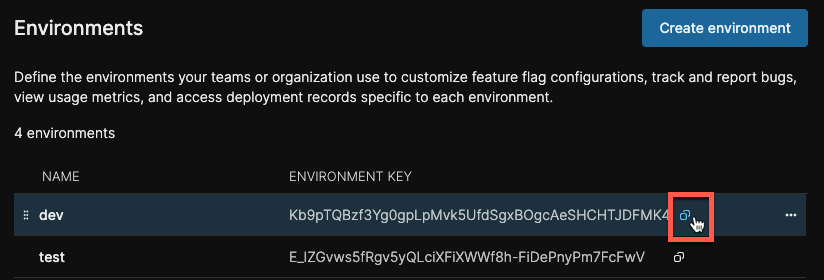
4. Customer and Location context
For some applications, you may need to provide additional context when evaluating feature flags, such as customer or location. The way this context is handled differs between the client-side and server-side SDKs.
Client-side SDK
In the client-side SDK, location is automatically fetched and set by the SDK during initialization, so you don't need to manually set it. This simplifies cases where the user's location is relevant for flag evaluation.
However, for other context variables, like customer, you can manually set the context:
const { Atono } = require('@atono-io/web-sdk');
const atono = Atono.fromEnvironmentKey('$ENV_KEY');
const featureFlags = await atono.getFeatureFlags();
// Set the customer context for this specific flag evaluation
await featureFlags.setContext({ customer: 'Virtual-Vintage' });
const amazingFeatureEnabled = featureFlags.getBooleanValue('amazing_feature_enabled', false);
if (amazingFeatureEnabled) {
console.log('Amazing feature is enabled π');
}
Since the user or location context doesnβt change often on the client-side, you only need to set this once when initializing the SDK.
React SDK
Set the evaluation context when configuring the in your Root component:
import { AtonoProvider } from '@atono-io/react-sdk';
function RootComponent() {
const flagEvaluationContext = { customer: 'Virtual-Vintage' };
return (
<AtonoProvider
environmentKey="<your-environment-key>"
evaluationContext={flagEvaluationContext}
>
<ChildComponent />
</AtonoProvider>
);
}
Server-side SDK
On the server-side, because different requests can come from different users, the evaluation context needs to be passed dynamically with each request.
Here's an example of how you might pass the context for a flag evaluation:
const { Atono } = require('@atono-io/server-sdk');
const atono = Atono.fromEnvironmentKey('$ENV_KEY');
const featureFlags = await atono.getFeatureFlags();
const evaluationContext = featureFlags.makeEvaluationContext({ customer: 'Virtual-Vintage' });
const amazingFeatureEnabled = featureFlags.getBooleanValue('amazing_feature_enabled', false, evaluationContext);
if (amazingFeatureEnabled) {
console.log('Amazing feature is enabled π');
}
You can optimize how to handle this evaluation context, depending on your application architecture. For example, you may want to cache the context for a session or create a new one for each request.
What about React server-side rendering?
We don't currently offer a React server-side SDK. If this is something you'd find valuable, share your thoughts with us in the #enhancements channel in the Atono Slack community!
Updated about 1 month ago